728x90
1. 배열과 링크드 리스트
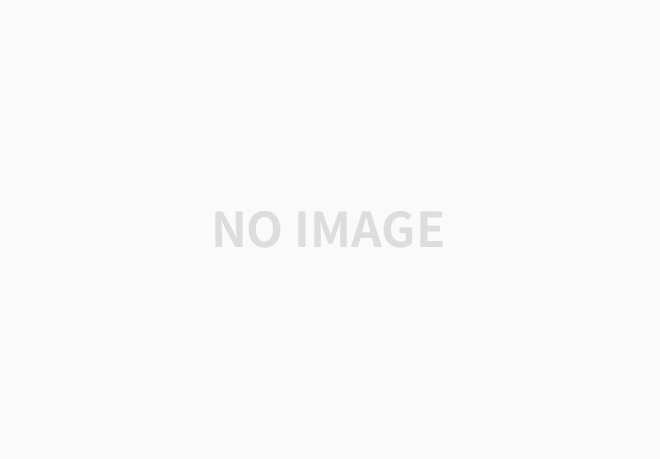
Python의 list :
링크드 리스트로 쓸 수도 있고, 배열로도 쓸 수 있게 만든 효율적인 자료구조
배열의 길이가 늘어나도 O(1) 의 시간 복잡도가 걸리도록 동적 배열 설계
2. 클래스
클래스 : 분류
객체 : 세상에 존재하는 유일무이한 사물
class Person:
pass # 여기서 pass 는 안에 아무런 내용이 없다는 의미입니다!
person_1 = Person()
print(person_1) # <__main__.Person object at 0x1090c76d0>
person_2 = Person()
print(person_2) # <__main__.Person object at 0x1034354f0>
생성자 :
객체를 생성할 때 데이터 넣어주기
내부적으로 원하는 행동 실행
(python에서 생성자 함수는 무조건 __init__)
self : 객체 자기 자신
method(메소드) : 클래스 내부의 함수
class Person:
def __init__(self):
print("hihihi", self)
person_1 = Person() # hihihi <__main__.Person object at 0x1067e6d60> 이 출력됩니다!
person_2 = Person() # hihihi <__main__.Person object at 0x106851550> 이 출력됩니다!
# self.name 에 param_name 을 저장해두겠다는 건
# 그 객체의 name 이라는 변수에 저장된다는 의미입니다!
class Person:
def __init__(self, param_name):
print("hihihi", self)
self.name = param_name
person_1 = Person("유재석") # hihihi <__main__.Person object at 0x1067e6d60> 이 출력됩니다!
print(person_1.name) # 유재석
person_2 = Person("박명수") # # hihihi <__main__.Person object at 0x106851550> 이 출력됩니다!
print(person_2.name) # 박명수
class Person:
def __init__(self, param_name):
print("hihihi", self)
self.name = param_name
def talk(self):
print("안녕하세요 저는", self.name, "입니다")
person_1 = Person("유재석") # hihihi <__main__.Person object at 0x1067e6d60> 이 출력됩니다!
print(person_1.name) # 유재석
person_1.talk() # 안녕하세요 저는 유재석 입니다
person_2 = Person("박명수") # # hihihi <__main__.Person object at 0x106851550> 이 출력됩니다!
print(person_2.name) # 박명수
person_2.talk() # 안녕하세요 저는 박명수 입니다
3. 링크드 리스트
3-1. append 함수 만들기,
링크드 리스트 모든 원소 출력하기
나의 풀이 : 아직 잘 못 풀겠음
튜터 님의 풀이 :
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self, value):
self.head = Node(value)
def append(self, value):
cur = self.head
while cur.next is not None:
cur = cur.next
cur.next = Node(value)
def print_all(self):
cur = self.head
while cur is not None :
print(cur.data)
cur = cur.next
linked_list = LinkedList(5)
linked_list.append(12)
linked_list.append(7)
linked_list.print_all()
'학습 내용 정리 > Algorithm' 카테고리의 다른 글
퀵 정렬 (Java7 이후 Arrays.sort()메서드의 Dual Pivot Quick Sort) (2) | 2024.04.02 |
---|---|
스파르타 코딩클럽 알고보면 알기 쉬운 알고리즘 1주차 (0) | 2023.06.26 |